Python Code Start Process in Suspended Mode
Table of Contents
I was working on a format string vulnerability challenge where the process would terminate quickly and was difficult to attach windbg for debugging. I wrote a small piece of code that will start the binary in suspended mode with arguments if required and attach the windbg You can resume the process using Process Hacker program. You can resume the process using Process Hacker program.
Note.: The windbg_path variable you need to adjust according to your system.
Steps to follow #
install pywin32 #
python -m pip install pywin32
Adjust the code and use #
After program is attached you can type g on windbg to let the debugger continue
import os
import win32process
import win32event
import win32api
bin_path = "C:\\binary_to_be_debugged.exe"
windbg_path = "C:\\Users\\User\\AppData\\Local\\Microsoft\\WindowsApps\\Microsoft.WinDbg_8wekyb3d8bbwe\\WinDbgX.exe"
def start_program_suspended(command):
# Create the process in a suspended state
startup_info = win32process.STARTUPINFO()
process_info = win32process.CreateProcess(
None,
" ".join(command),
None,
None,
False,
win32process.CREATE_SUSPENDED,
None,
None,
startup_info
)
return process_info
def attach_windbg(pid):
if pid:
# command to attach windbg to the process
windbg_command = f"{windbg_path} -p {pid}"
# Start Windbg and attach it to the process
os.system(windbg_command)
else:
print("\nProcess terminated too quickly. Restarting the process.")
def resume_process(process_info):
# Resume the suspended process
win32process.ResumeThread(process_info[1])
def sploit():
Argument = "ABCD"
command = [bin_path] + [Argument]
# Start the program in a suspended state
process_info = start_program_suspended(command)
# Get the process ID (PID)
pid = process_info[2]
# Attach windbg to the suspended process
attach_windbg(pid)
# Resume the process
resume_process(process_info)
# Wait for the process to complete
win32event.WaitForSingleObject(process_info[0],win32event.INFINITE)
# Close handles
win32api.CloseHandle(process_info[0])
win32api.CloseHandle(process_info[1])
if __name__ == '__main__':
sploit()
Process Hacker #
You can resume the process after the program is successfully attached to windbg by right click and click on Resume as showing on screenshot below.
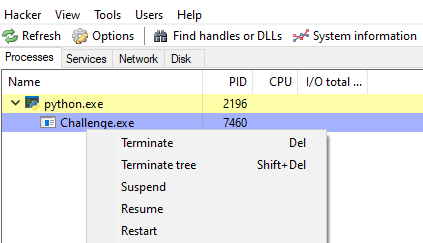